需要实现商品服务的这4个功能:
1.先从商品api文档入手,查看商品结构,如下:
请求方式:GET 请求URL:/product/list 返回参数: { "code": 0, "msg": "成功", "data": [ { "name": "热榜", "type": 1, "foods": [ { "id": "123456", "name": "皮蛋粥", "price": 1.2, "description": "好吃的皮蛋粥", "icon": "http://xxx.com", } ] }, { "name": "好吃的", "type": 2, "foods": [ { "id": "123457", "name": "慕斯蛋糕", "price": 10.9, "description": "美味爽口", "icon": "http://xxx.com", } ] } ] } |
2.新建数据库
3.新建类目、商品表
-- 商品 create table `product_info` ( `product_id` varchar(32) not null, `product_name` varchar(64) not null comment '商品名称', `product_price` decimal(8,2) not null comment '单价', `product_stock` int not null comment '库存', `product_description` varchar(64) comment '描述', `product_icon` varchar(512) comment '小图', `product_status` tinyint(3) DEFAULT '0' COMMENT '商品状态,0正常1下架', `category_type` int not null comment '类目编号', `create_time` timestamp not null default current_timestamp comment '创建时间', `update_time` timestamp not null default current_timestamp on update current_timestamp comment '修改时间', primary key (`product_id`) ); INSERT INTO `product_info` (`product_id`, `product_name`, `product_price`, `product_stock`, `product_description`, `product_icon`, `product_status`, `category_type`, `create_time`, `update_time`) VALUES ('157875196366160022','皮蛋粥',0.01,39,'好吃的皮蛋粥','//fuss10.elemecdn.com/0/49/65d10ef215d3c770ebb2b5ea962a7jpeg.jpeg',0,1,'2017-03-28 19:39:15','2017-07-02 11:45:44'), ('157875227953464068','慕斯蛋糕',10.90,200,'美味爽口','//fuss10.elemecdn.com/9/93/91994e8456818dfe7b0bd95f10a50jpeg.jpeg',1,1,'2017-03-28 19:35:54','2017-04-21 10:05:57'), ('164103465734242707','蜜汁鸡翅',0.02,982,'好吃','//fuss10.elemecdn.com/7/4a/f307f56216b03f067155aec8b124ejpeg.jpeg',0,1,'2017-03-30 17:11:56','2017-06-24 19:20:54'); |
4.在IDEA新建springCloud项目-商品服务
修改版本,和之前建的eureka项目版本一致,修改完记得刷新:
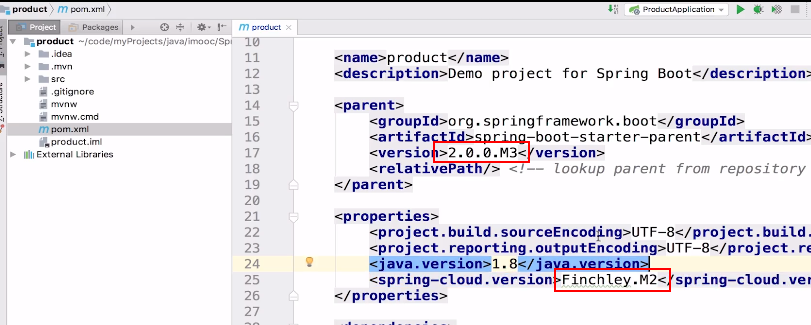
删除掉不需要的文件:
5.把商品服务注册到eureka上去,启动项目
将application.properties修改为application.yml
记得加上这个注解,再启动项目:
打开8761的eureka,有如下页面则成功将商品服务注册到8761的eureka上:
6.商品业务实现
在pom.xml添加spring-boot-starter-data-jpa和mysql-connector-java依赖,且刷新:
在application.yml配置数据库信息
新建ResultVO.java——http请求返回的最外层对象
ResultVO.java
/** * http请求返回的最外层对象 */ public class ResultVO<T> {/** * 错误码 */ private Integer code;/** * 提示信息 */ private String msg;/** * 具体内容 */ private T data; } |
测试类,不需每次都去拷贝这2个注解了,,直接用@Component注解,且继承公共测试类:
新建ResultVOUtil.java
ResultVOUtil.java
public class ResultVOUtil { public static ResultVO success(Object object) { ResultVO resultVO = new ResultVO(); resultVO.setData(object); resultVO.setCode(0); resultVO.setMsg("成功"); return resultVO; } } |
1) model 配置表对应的实体类,类名、字段最好和表中一致
a.使用这个插件,可以省略getter/setter方法
在pom.xml加入lombok依赖 还需要在idea下载这个插件 只需要在实体类加上@Data注解,就可以不用在实体类生成getter/setter方法了 |
新建实体类ProductInfo.java、ProductCategory.java
ProductInfo.java
//@Table(name = "T_proxxx") public class ProductInfo { private String productId; /** 名字. */ private String productName;/** 单价. */ private BigDecimal productPrice;/** 库存. */ private Integer productStock;/** 描述. */ private String productDescription;/** 小图. */ private String productIcon;/** 状态, 0正常1下架. */ private Integer productStatus;/** 类目编号. */ private Integer categoryType;private Date createTime; private Date updateTime; } |
ProductCategory.java
@Data @Entity public class ProductCategory {@Id @GeneratedValue private Integer categoryId;/** 类目名字. */ private String categoryName;/** 类目编号. */ private Integer categoryType;private Date createTime; private Date updateTime; } |
2) dao
接口1:查询所有在架的商品——新建dao,ProductInfoRepository.java
可以单元测试一下:
接口2:查询类目type列表——新建dao,ProductCategoryRepository.java
3) service
新建ProductService.java、ProductServiceImpl.java
ProductService.java
public interface ProductService { /** * 查询所有在架商品列表 */ List<ProductInfo> findUpAll();/** * 查询商品列表 * @param productIdList * @return */ List<ProductInfoOutput> findList(List<String> productIdList); } |
ProductServiceImpl.java
@Service public class ProductServiceImpl implements ProductService {@Autowired private ProductInfoRepository productInfoRepository;@Override public List<ProductInfo> findUpAll() { return productInfoRepository.findByProductStatus(ProductStatusEnum.UP.getCode()); }@Override public List<ProductInfoOutput> findList(List<String> productIdList) { return productInfoRepository.findByProductIdIn(productIdList).stream() .map(e -> { ProductInfoOutput output = new ProductInfoOutput(); BeanUtils.copyProperties(e, output); return output; }) .collect(Collectors.toList()); } } |
新建CategoryService.java、CategoryServiceImpl.java
CategoryService.java
public interface CategoryService { List<ProductCategory> findByCategoryTypeIn(List<Integer> categoryTypeList); } |
CategoryServiceImpl.java
@Service public class CategoryServiceImpl implements CategoryService {@Autowired private ProductCategoryRepository productCategoryRepository;@Override public List<ProductCategory> findByCategoryTypeIn(List<Integer> categoryTypeList) { return productCategoryRepository.findByCategoryTypeIn(categoryTypeList); } } |
4) vo
新建ProductVO.java、ProductInfoVO.java
ProductVO.java
@Data public class ProductVO {@JsonProperty("name") //@JsonProperty返回给前端的字段 private String categoryName;@JsonProperty("type") private Integer categoryType;@JsonProperty("foods") List<ProductInfoVO> productInfoVOList; } |
ProductInfoVO.java
@Data public class ProductInfoVO {@JsonProperty("id") private String productId;@JsonProperty("name") private String productName;@JsonProperty("price") private BigDecimal productPrice;@JsonProperty("description") private String productDescription;@JsonProperty("icon") private String productIcon; } |
5) controller
新建ProductController.java
ProductController.java
@RestController @RequestMapping("/product") public class ProductController {@Autowired private ProductService productService;@Autowired private CategoryService categoryService;/** * 1. 查询所有在架的商品 * 2. 获取类目type列表 * 3. 查询类目 * 4. 构造数据 */ @GetMapping("/list") public ResultVO<ProductVO> list() { //1. 查询所有在架的商品 List<ProductInfo> productInfoList = productService.findUpAll();//2. 获取类目type列表 List<Integer> categoryTypeList = productInfoList.stream() .map(ProductInfo::getCategoryType) .collect(Collectors.toList());//3. 从数据库查询类目 List<ProductCategory> categoryList = categoryService.findByCategoryTypeIn(categoryTypeList);//4. 构造数据 List<ProductVO> productVOList = new ArrayList<>(); for (ProductCategory productCategory: categoryList) { ProductVO productVO = new ProductVO(); productVO.setCategoryName(productCategory.getCategoryName()); productVO.setCategoryType(productCategory.getCategoryType());List<ProductInfoVO> productInfoVOList = new ArrayList<>(); for (ProductInfo productInfo: productInfoList) { if (productInfo.getCategoryType().equals(productCategory.getCategoryType())) { ProductInfoVO productInfoVO = new ProductInfoVO(); BeanUtils.copyProperties(productInfo, productInfoVO); productInfoVOList.add(productInfoVO); } } productVO.setProductInfoVOList(productInfoVOList); productVOList.add(productVO); }return ResultVOUtil.success(productVOList); }/** * 获取商品列表(给订单服务用的) * * @param productIdList * @return */ @PostMapping("/listForOrder") public List<ProductInfoOutput> listForOrder(@RequestBody List<String> productIdList) { try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } return productService.findList(productIdList); }@PostMapping("/decreaseStock") public void decreaseStock(@RequestBody List<DecreaseStockInput> decreaseStockInputList) { productService.decreaseStock(decreaseStockInputList); } } |
7.访问成功